Python使用中面向对象的语言,支持继承、多态;
定义一个Person类:
复制代码 代码如下:
>>> class Person:
... def sayHello(self):
... print('hello')
...
>>> Person.sayHello(None)
hello
>>> Person().sayHello()
hello
可以修改Person的类方法
复制代码 代码如下:
>>> def hack_sayHello(obj):
... print('...hello')
...
>>>
>>> Person.sayHello = hack_sayHello
>>> Person.sayHello(None)
...hello
>>> Person().sayHello()
...hello
>>> sayHello = Person().sayHello
>>> sayHello()
...hello
Person().sayHello也是一个函数,可以赋值给变量,并可以直接调用;
复制代码 代码如下:
>>> Person.sayHello is Person().sayHello
False
>>> Person.sayHello == Person().sayHello
False
Person.sayHello与Person().sayhello并不是同一个对象,直觉上,Person().sayHello关联(绑定)了一个Person实例,而Person.sayHello是一个类方法;
self参数事实上正是方法和函数的区别:方法将它们的第一个参数绑定到所属的实例上,因此这个参数可以不必提供;
复制代码 代码如下:
>>> class Person:
... name = 'unkown'
... def sayHello(self):
... print('i\'m ' + name)
...
>>>
>>> Person.sayHello(None)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 4, in sayHello
NameError: name 'name' is not defined
>>> p = Person()
>>> p.name = 'wyj'
>>> p.sayHello()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 4, in sayHello
NameError: name 'name' is not defined
可见,Python在解析变量时,默认从local scope/global scope中查找;
复制代码 代码如下:
>>> class Person:
... name = 'unkown'
... def sayHello(self):
... print('i\'m ' + self.name)
...
>>>
>>> Person.sayHello(None)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 4, in sayHello
AttributeError: 'NoneType' object has no attribute 'name'
>>> p = Person()
>>> p.name = 'wyj'
>>> p.sayHello()
i'm wyj
访问成员都要通过self,假如以包含name属性的对象调用Person.sayHello(obj),是否可以呢?
复制代码 代码如下:
>>> class Cat:
... name = 'huanhuan'
...
>>> Person.sayHello(Cat())
i'm huanhuan
可以,Python并不限制必须用相同类的实例对象作为参数调用类方法(貌似Python的类机制类似Javascript);
访问控制
Python并不直接支持私有方访问,而是要靠程序员自己把握。
不过,可以在属性名称前加上双下划线而给其私有访问能力(对外不可见);
复制代码 代码如下:
>>> class Person:
... def __private_method(self):
... print('private')
... def test(self):
... self.__private_method()
...
>>> Person().test()
private
>>> Person().__private_method()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'Person' object has no attribute '__private_method'
实际上,以上下划线打头的方法都有一个_ClassName__methodName的方法
复制代码 代码如下:
>>> Person._Person__private_method
<function Person.__private_method at 0x7fed431a2d90>
调用
复制代码 代码如下:
>>> Person._Person__private_method(None)
private
总之,Python并不能阻止从类外进行方法调用;
类属性以及对象属性
首先,可以为类添加属性,新对象将得到属性的一份拷贝
复制代码 代码如下:
>>> Person.age = 3
>>> Person().age
3
>>> Person.age = 4
>>> Person().age
4
>>> p = Person()
>>> Person.age = 31
>>> p.age
31
对类属性的修改,反映到了先前生成的对象的属性上,这说明类属性和对象的属性共享一个值;
复制代码 代码如下:
>>> p.age = 34
>>> p.age
34
>>> Person.age
31
>>> Person.age = 99
>>> p.age
34
而一旦对对象的属性的修改,对象属性就拥有了自己的值,并不会反映到类属性上,而对类属性的修改,也不再反映到该对象的属性上;
这种行为与Javascript类似
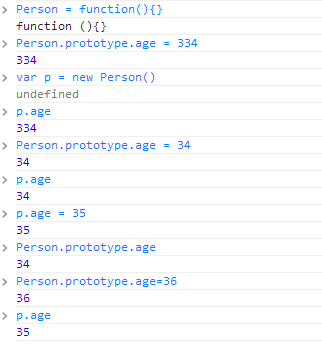
jsjbwy